-
0 Comments
Discover the power of Django, the Python web framework that simplifies development. Learn what it is, why it’s the right choice for your projects, and its key features. From installation to creating your first app and models, this guide will walk you through the essentials of Django development.
Django is a powerful and popular Python framework for building web applications. It provides a robust structure and tools to accelerate development, making it a great choice for both beginners and experienced developers.
What is Django Python?
Django Python is a free and open-source project, high-level full-stack web framework written in python. What is MVT — The MVT (Model-View-Template) pattern shapes the architecture and working part of a web application.
FeaturesRapid development: Django is known for its rapid development capabilities and “batteries included” philosophy, which means that it comes with a large number of features built-in.
Why Choose Django Python?
Speed of Development: Django help in speeding up the development and completion process webapp using its built-in tools.
Scalability: Django is easily scalable to high traffic and complex applications.
Security: Django offers built-in security mechanism to make sure your applications are safe from common vulnerabilities.
Active Community: Django has a wide community base and provides robust documentation, tutorials as well support.
Versatility: Django can be applied to build a different types of web applications from easy websites through complex enterprise solutions.
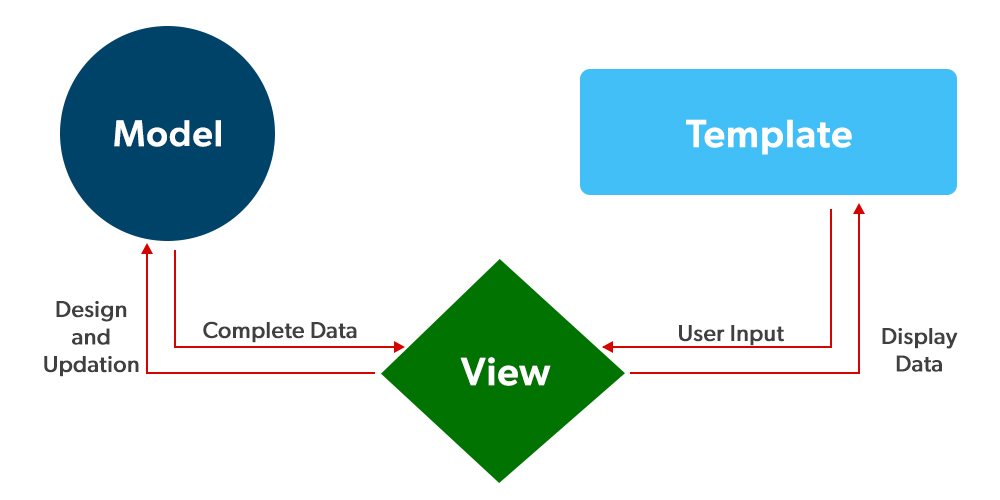
Key Features of Django Python
MVT Architecture: M in Django stands for the model, which is responsible for maintaining your data.
Model: It represent data of the application.
View: Responsible for process the requests and generate response(s) in logics.
Template: Presentation Layer of your Application.
Django ORM: Having a high-level database interface where we can query, insert, update and delete data there are some tweaks to make the code simple but powerful.
Template Engine: template engine provides a way of generating HTML content with the use of simple syntax.
Authentication and Authorization: Django has built-in tools for user authentication, creating customisable user accounts, and defining permissions. With Django comes an admin interface that you can use to interact with the data in your application from a web-based alternative.
Caching: Different caching mechanisms Django supports to increase performance and reduce database load.
Django deployment: One can easily deploy Django on different platforms such as Heroku, AWS, and DigitalOcean.
Django is known for “Batteries included”: Django comes with a rich set of built-in libraries and features — ORM, authentication/authorization, caching etc.
Scalperable: Django is designed to work best for high traffic, complex applications and serves as an excellent candidate when it comes to building large-scale project.
Safe and secure: With Django, it’s rather easy to build a safe platform by limiting common web problems such as SQL intrusion, XSS protection or CSRF.
Community and support: Django has a huge community that covers an extensive list of documentations, tutorials to help you get started.
Django: Django is a high-level Python web framework that encourages rapid development and clean, pragmatic design.
Versatility: Due to its versatility, from simple websites to complex enterprise applications can be developed using django.
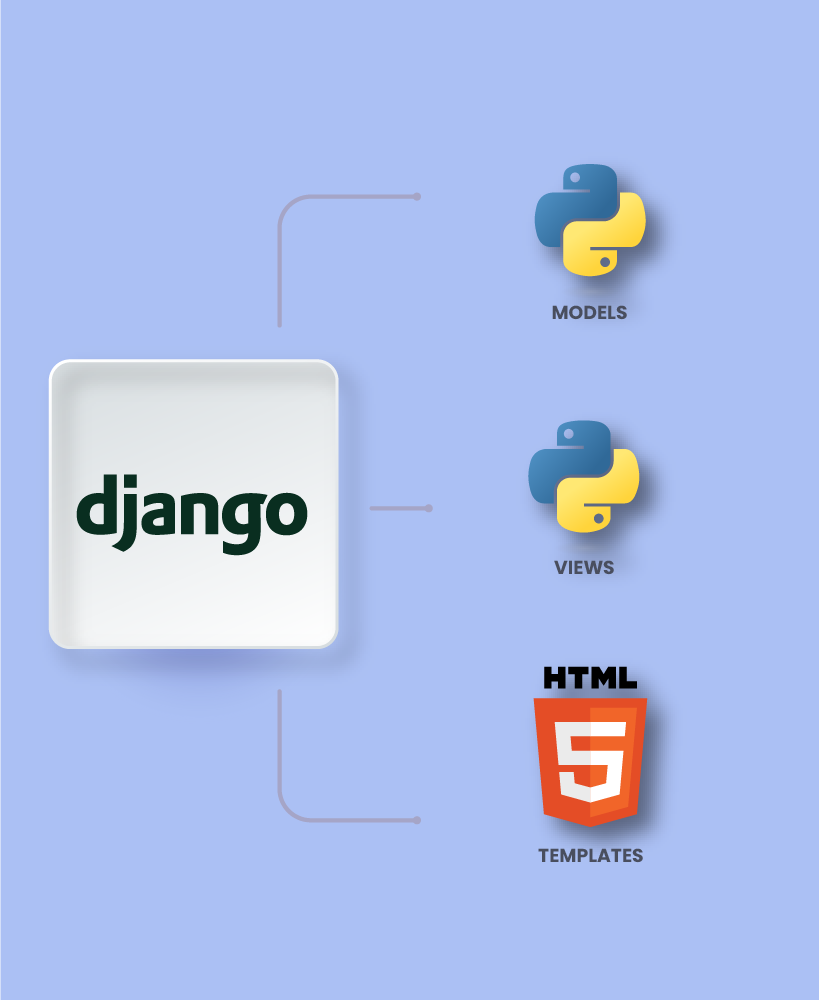
Django Project Structure
Project Directory:
The project directory is the root of your application. It contains various subdirectories, including settings, urls, and apps.
Settings.py:
The settings.py file configures your Django project. It defines database connections, installed apps, and other project-specific settings.
URLs.py:
This file maps URLs to views in your application. It defines the routing logic, determining which view should handle each URL request.
More on these topics before starting with Django Python
Django REST Framework (DRF)
Django REST framework is a powerful and flexible toolkit for building Web APIs using the Django web_framework. It provides features like:
Serializers: Python data structure <-> JSON, XML or other format
Viewsets: Allows you to quickly build API views.
Excessive level Vs Preconditions such as authentication and Permissions: Configure the preconditions by enabling or disabling them like implementing Api and permissions.
iOS Push Notifications: IOS Local Notification, UIPasteboard Android Alarm Manager & shared preferences (Android) Using instrument for by simulating the app only with 60% battery leftPagination Handle large dataset using pagination.
Filtering : It enables clients and third-party applications to filter collection data based on given criteria.
Django Channels
If you are not familiar with it, Django Channels is a little bit on the low level side as far as frameworks go and allows us to build real-time applications in what I think of one step up from socket.io for express.js. It makes you capable to manage WebSocket connections, HTTP/2,… etc:async protocols.
Django Templates
Django has a template engine that allows you to generate HTML dynamically.
Key features include:
Variables: View and use the variables in your templates.
Tags: Conditionals (logic & control flow) in your templates
Filters: Filter and Format Variables.
Inherit: Create templates on base of the parent template, launching them with a separate view.
Django Admin
Django admin is an automatically-generated user interface for managing your site’s data. It can be adjusted to fit your own needs
Django Deployment
Django can be installed onvzew wide range of platforms:
Heroku: It is an awesome cloud platform to deploy any kind of web application.
Amazon Web Services (AWS): AWS has numerous services that you can use to deploy Django apps including EC2, S3 and Elastic Beanstalk.
DigitalOcean: Cloud infrastructure provider offering cheap Virtual Private Servers to host Django apps in the wild.
Getting Started with Django Python
Download the Django Framework Click Here:
- Install Django: To get started with Django Python, you’ll need to have Python installed on your system. You can download the latest version of Python from the official website
- Create a New Project: Create a new Django project using the “django-admin startproject” command. This will create a new directory named myproject that contains the basic structure of a Django project.
- Create an App: Create a new Django app using the “django-admin startapp” command.
- Configure Your Project: Add your app to the INSTALLED_APPS setting in your project’s settings.py file.
- Run the Development Server: Start the development server using the “python manage.py runserver”command.
Creating Models in Django Python
Models in Django Python represent the data structure of your application. They are defined using Python classes and are mapped to database tables.
Here’s a simple example of a BlogPost model:
Python from django.db import models
class BlogPost(models.Model):
title = models.CharField(max_length=200)
content = models.TextField()
Django Python in Action: A Simple Example
Here’s a simple Django project that creates a basic web page:
Python
# myapp/views.py
from django.shortcuts import render
def index(request):
return render(request, ‘myapp/index.html’, {‘name’: ‘Django’})
# myapp/templates/myapp/index.html
<!DOCTYPE html>
<html>
<head>
<title>Django Example</title>
</head>
<body>
<h1>Hello, {{ name }}!</h1>
</body>
</html>
Creating Templates in Django Python
Templates in Django Python are used to define the structure and presentation of your application’s user interface. They are written in a simple template language that allows you to embed Python code within HTML.
Here’s a simple example of a template that displays a list of blog posts:
HTML
<!DOCTYPE html>
<html>
<head>
<title>My Blog</title>
</head>
<body>
<h1>My Blog</h1>
<ul>
{% for blog_post in blog_posts %}
<li>
<h2>{{ blog_post.title }}</h2>
<p>{{ blog_post.content }}</p>
</li>
{% endfor %}
</ul>
</body>
</html>
Conclusion
Django Python is a powerful and versatile web framework that can help you build robust and scalable web applications. With its rich feature set, large community, and ease of use, Django is an excellent choice for developers of all levels.